Listening to a message broker with php
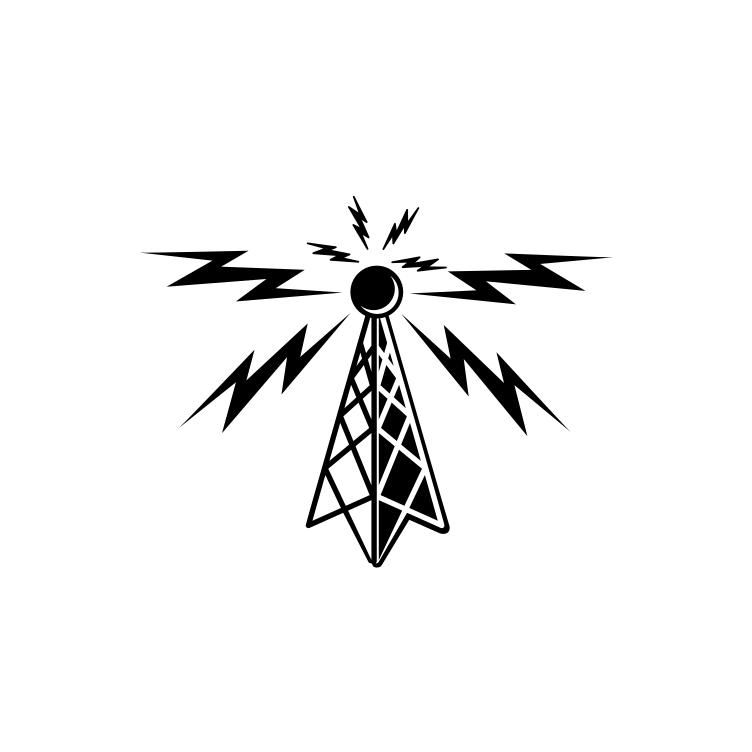
Integrating PHP with Message Brokers: An Actionable Guide
In the world of distributed systems and microservices, message brokers have become an integral component. They facilitate communication between different parts of a system, ensuring data gets where it needs to go. PHP, as a popular backend language, can be integrated with message brokers to listen to messages and perform actions based on them. In this blog post, we'll explore how to do just that.
What is a Message Broker?
A message broker acts as an intermediary for messaging between different parts of a system. It can receive messages from a producer service, store them, and deliver them to consumer services when appropriate. Examples include RabbitMQ, Apache Kafka, and ActiveMQ.
Why integrate PHP with a Message Broker?
Imagine you have an e-commerce website built using PHP. When a user places an order, you might want to notify multiple services - billing, shipping, and inventory, for example. A message broker can distribute this message to all these services, ensuring no service is overwhelmed with direct requests.
Listening to a Message Broker with PHP: A RabbitMQ Example
For this guide, we'll use RabbitMQ, a widely-used open-source message broker, and the PHP AMQP library.
Prerequisites
- A running instance of RabbitMQ. You can install it following the official guide.
- PHP and Composer installed.
Steps:
1. Install the PHP AMQP Library:
Using Composer, install the php-amqplib/php-amqplib
package:
composer require php-amqplib/php-amqplib
2. Set up Connection:
Create a new PHP script and set up a connection to RabbitMQ:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpAmqpLib\Connection\AMQPStreamConnection;
$connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest');
$channel = $connection->channel();
Here, we're connecting to a local RabbitMQ instance with the default credentials.
3. Declare a Queue:
Before we can listen to messages, we need to declare a queue:
$queueName = 'test_queue';
$channel->queue_declare($queueName, false, false, false, false);
4. Listen to Messages and Perform Action:
Now, let's listen to messages and print them out:
echo "Waiting for messages. To exit press CTRL+C\n";
$callback = function ($msg) {
echo 'Received: ', $msg->body, "\n";
// Here, you can perform any action based on the message content.
// For instance, sending an email, updating a database, etc.
};
$channel->basic_consume($queueName, '', false, true, false, false, $callback);
while ($channel->is_consuming()) {
$channel->wait();
}
Run your PHP script, and it will start listening to messages sent to the 'test_queue' queue. Whenever a message is received, it will print it out and perform any defined actions within the callback.
5. Cleanup:
After you're done, it's essential to close the channel and connection:
$channel->close();
$connection->close();
Conclusion:
Message brokers are a powerful way to decouple components in a distributed system. By integrating PHP with RabbitMQ, you can easily set up event-driven architectures where PHP services listen to messages and act on them. While we've used RabbitMQ in this example, the principles are similar for other message brokers. Dive in, explore, and let your systems communicate seamlessly.